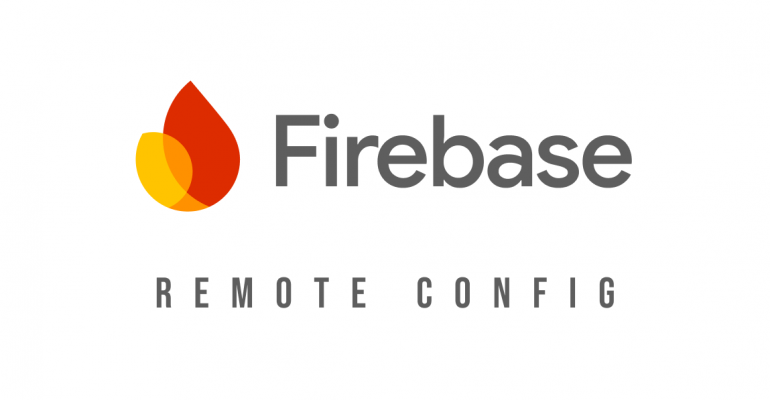
Firebase Remote Config
Von Katharina Wurm am 25.11.2024
Recently, I did a workshop on Firebase and Cloud Firestore during the course of my studies. To make sure I had enough material to fill one third of the lecture, I prepared an extra topic: Firebase Remote Config. However, the other workshop contents (which I described in the linked article) filled the time well, and the extra preparation was unused. Thus, I thought: why let the time spent on preparing this extra topic go to waste? So, here it is, the part of my workshop, which I did not get to teach, as a normal blog article. If you are interested, keep reading. 😉
What is Firebase Remote Config?
Remote Config is a Firebase service which allows you to change your app’s behaviour and appearance without having to publish a downloadable app update. You can set key-value pairs in the Firebase Console, which are fetched and then applied by your app.
For example, you could use Remote Config to dynamically change your app’s colour theme, like I will show you now.
Example Implementation
Step 1: Preparation
This implementation builds upon the example app we worked on during the workshop on Firebase and Cloud Firestore. Check out the article to find out how to set up a Firebase project and connect it with your React app.
Within the workshop’s starter project, I had already prepared the theme to be changeable with one boolean value. Our goal now is to change the code so that the boolean value is not hard-coded, but instead retrieved from Remote Config.
First, let’s look at the relevant starter project’s code. I used Material UI for theming (https://mui.com/material-ui/customization/theming/). At the time of writing this, I was pretty new to MUI within React, so it is possible that my code could be improved. However, the point of this article is to show you how you could use Firebase Remote Config, not how to use MUI for theming, after all. 🙂
src/theme.ts
Within the theme.ts file, I determine the theme’s primary and secondary colour based on the „alternativeTheme“ boolean value.
import {createTheme} from "@mui/material";
import {useEffect, useState} from "react";
const defaultPrimaryColour = '#3f51b5';
const alternativePrimaryColour = '#52a681';
const defaultSecondaryColour = '#f50057';
const alternativeSecondaryColour = '#5a3fb5';
export const useCustomTheme = () => {
const [primaryColour, setPrimaryColour] = useState(defaultPrimaryColour);
const [secondaryColour, setSecondaryColour] = useState(defaultSecondaryColour);
useEffect(() => {
const alternativeTheme = false // TODO: get this value from remote config
setPrimaryColour(alternativeTheme ? alternativePrimaryColour : defaultPrimaryColour);
setSecondaryColour(alternativeTheme ? alternativeSecondaryColour : defaultSecondaryColour)
}, []);
return createTheme({
palette: {
mode: 'light',
primary: {
main: primaryColour,
},
secondary: {
main: secondaryColour,
},
},
});
};
src/main.tsx
This custom theme is then called within the main.tsx file.
import { StrictMode } from 'react'
import { createRoot } from 'react-dom/client'
import './index.css'
import App from './App.tsx'
import {ThemeProvider} from "@mui/material";
import {useCustomTheme} from "./theme.ts";
const Root = () => {
const theme = useCustomTheme();
return (
<ThemeProvider theme={theme}>
<App />
</ThemeProvider>
);
};
createRoot(document.getElementById('root')!).render(
<StrictMode>
<Root />
</StrictMode>,
)
Simple enough, right? Let’s get to the fun part!
Step 2: Create Remote Config
- Choose “Remote Config” in the “Run” dropdown list within the menu on the left side of the Firebase Console.
- Click on „Create Configuration“.
- It will then ask you to create your first parameter, your first key-value pair.
- For this example implementation, I chose to call it „alternativeTheme“. Select „Boolean“ as the data type and set its default value to „false“.
- Click „save“.
- However, simply saving the parameter did not actually distribute it to our app. Changes to Remote Config parameters always need to be explicitly „published“.
- Thus, click on „Publish changes“ at the top of the page.
Step 3: Coding Time! / Make the React project fetch the Remote Config parameter
First, we have to update the Firebase Config file. This is the file in which you put your Firebase secrets to connect the React app with the Firebase project (see workshop on Firebase and Cloud Firestore).
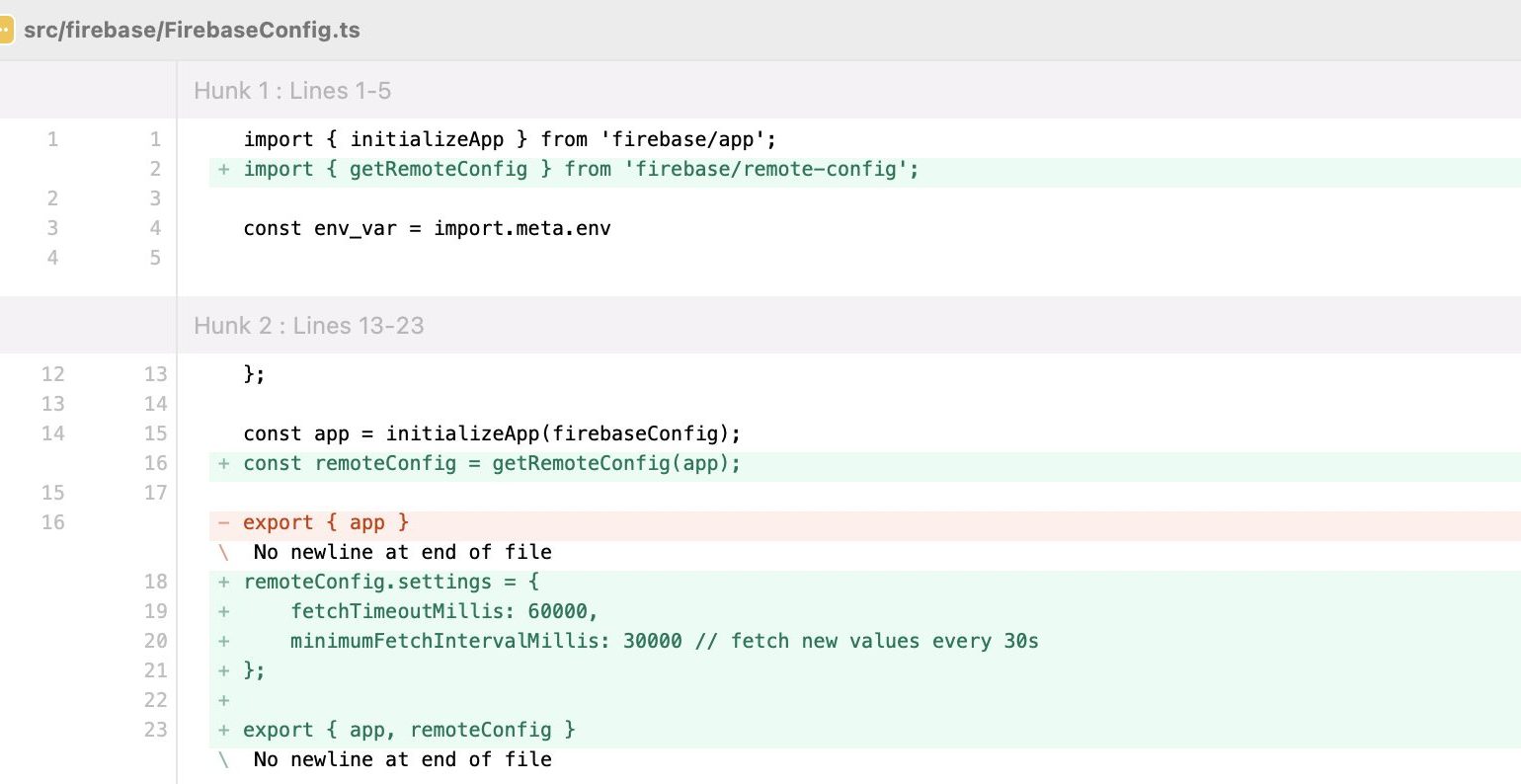
Something to point out here is line 20, where we set the „minimumFetchIntervalMillis“. This tells our app how often to check the Remote Config for new values. Once you move to production, this value should be relatively high, so you do not send too many fetch requests too frequently. I believe that currently, it defaults to 43200000ms (= 12 hours). However, during development, we do not want to wait that long to see a change in the Firebase Console go into effect. Thus, for this project, I have set it to 30s for now.
Finally, we have to adjust the theme.ts file to fetch the „alternativeTheme“ value from Remote Config.
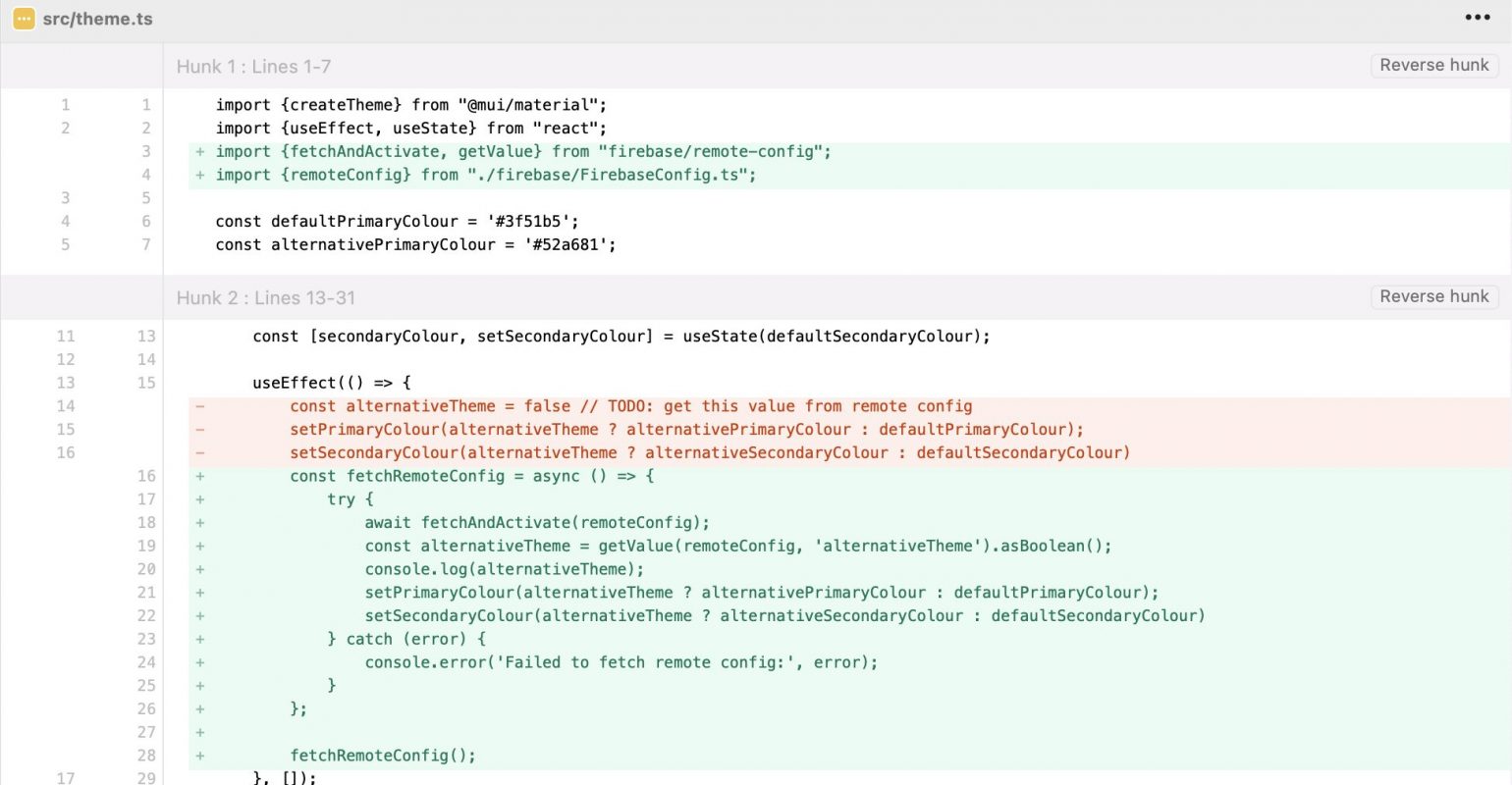
As you can see, Firebase provides us with some handy functions we can use to „fetchAndActivate“ the Remote Config and to get the boolean value. Since something can go wrong while fetching the value, we use a try-catch to handle any potential errors appropriately.
You can now try to go into the Firebase Console and change the „alternativeTheme“ value to „true“. Make sure to save and publish the changes, and if everything went well, your app’s colours will change. PS: if it does not happen right away, wait 30s like we have set, and refresh the page.
Conclusion
That was it! Pretty cool, right? You can use Firebase Remote Config for a variety of use cases, from changing small texts in your app to unlocking hidden functionalities. I am excited to see what you will come up with.
This article not affiliated with, supported, or endorsed by Firebase or its parent company, Google. The content shared in the article reflects my own experiences and understanding of the Firebase platform.
The comments are closed.